Have you ever needed to generate a PDF from HTML using C#? If so, you may have found that there are not many libraries that can easily do this. However, there is one library that stands out from the rest: Puppeteer Sharp.
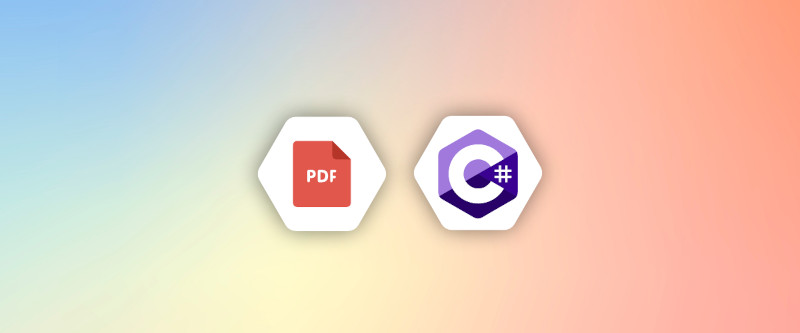
Puppeteer Sharp is a C# port of the popular Puppeteer library, which is used for headless Chrome automation. Puppeteer Sharp takes the best of Puppeteer and brings it to .NET(or .Net Core). One of the many things that Puppeteer can do is generate PDFs from HTML.
PDFs are a great way to share documents, as they can be viewed on any device and are difficult to modify. This makes them ideal for things like invoices, packing slips, and other documents where you don’t want the recipient to be able to make changes.
HTML templates are a great way to create PDFs, as they allow you to easily control the layout of the document. Plus, using a template ensures that your PDF will always look consistent, even if the data changes.
In this article, we will see how to use Puppeteer Sharp to generate PDFs from HTML templates.
1. Generate PDF with Puppeteer Sharp
First, we need to install the Puppeteer Sharp
NuGet package in your dotnet core project. We can do this by using the below command:
dotnet add package PuppeteerSharp
Once the package is installed, we can start writing some code. We need to import the following namespaces:
using PuppeteerSharp;
using System.IO;
We also need to make sure that we are using the async
keyword, as Puppeteer Sharp is an asynchronous library.
Next, we need to define our HTML template. For this example, we will just use a very simple template:
<html>
<body>
<h1>Invoice</h1>
<p>
Invoice #: 123<br />
Date: 1/1/2020
</p>
<table>
<tr>
<th>Item</th>
<th>Price</th>
</tr>
<tr>
<td>Widget</td>
<td>$10.00</td>
</tr>
<tr>
<td>Gadget</td>
<td>$20.00</td>
</tr>
</table>
<p>
Total: $30.00
</p>
</body>
</html>
Now that we have our template, we can write the code to generate the PDF:
var html = File.ReadAllText("invoice.html");
var pdfOptions = new PuppeteerSharp.PdfOptions();
pdfOptions.Format = PuppeteerSharp.PaperFormat.A4;
using (var browser = await Puppeteer.LaunchAsync(new LaunchOptions
{
Headless = true,
ExecutablePath = "CHROME_BROWSER_PATH"
}))
{
using (var page = await browser.NewPageAsync())
{
await page.SetContentAsync(html);
await page.PdfAsync("invoice.pdf", pdfOptions);
}
}
Let’s break down what this code is doing. First, we are reading in the HTML template using the File.ReadAllText
method. Next, we are creating a new PdfOptions
object. This object allows us to set the paper size and orientation.
We are then using the PuppeteerSharp.LaunchAsync
method to launch a new headless Chrome instance. We are using the async
keyword here so that we can await
the async
methods that PuppeteerSharp
provides.
Once we have a new page, we are setting the content of the page to our HTML template using the SetContentAsync
method. Finally, we are using the PdfAsync
method to generate the PDF and save it to the invoice.pdf file.
And that’s it! We now have a simple way to generate PDFs from HTML templates using C#.
Below is the full working code for this example:
using PuppeteerSharp;
using System.IO;
namespace PdfFromHtml
{
class Program
{
static async Task Main(string[] args)
{
var html = File.ReadAllText("./invoice.html");
var pdfOptions = new PuppeteerSharp.PdfOptions();
using (var browser = await Puppeteer.LaunchAsync(new LaunchOptions
{
Headless = true,
ExecutablePath = "CHROME_BROWSER_PATH"
}))
{
using (var page = await browser.NewPageAsync())
{
await page.SetContentAsync(html);
await page.PdfAsync("invoice.pdf", pdfOptions);
}
}
}
}
}
Run the code using the below command:
dotnet run
The following is the generated PDF
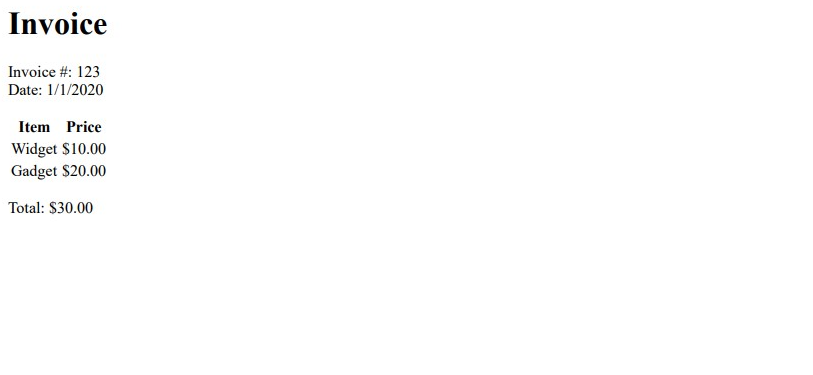
2. Generate PDF with APITemplate.io
APITemplate.io is a cloud-based API that allows you to generate PDFs from templates. It is a subscription-based service that is scalable and easy to use.
2.1 Why use APITemplate.io?
There are several reasons why you would want to use APITemplate.io to generate PDFs. Below are some of the benefits:
i. Cloud-based API
APITemplate.io is a cloud-based API, which means you don’t need to set up any infrastructure or servers. All you need is an internet connection and you’re good to go.
ii. No need to set up Infrastructure or Servers
With APITemplate.io, you can integrate with our API in a matter of an hour, and everything is taken care of for you in the cloud! There is no need to worry about setting up your own servers for your PDF generation.
iii. Subscription-based
APITemplate.io is a subscription-based service, which means you only pay for what you use. Our pricing is transparent and goes by monthly or annual subscription, there are no upfront costs or contracts and you can cancel at any time.
If you need to generate more PDFs, you can simply upgrade your subscription.
iv. Scalable
APITemplate.io is scalable and we support parallel asynchronous or synchronous PDF conversions. With asynchronous support, we will notify you once the PDFs are ready.
2.2 Getting Started with APITemplate.io
Getting started with APITemplate.io is easy. Follow the steps below to get started:
Step 1. Go to APITemplate.io and sign up for an account.
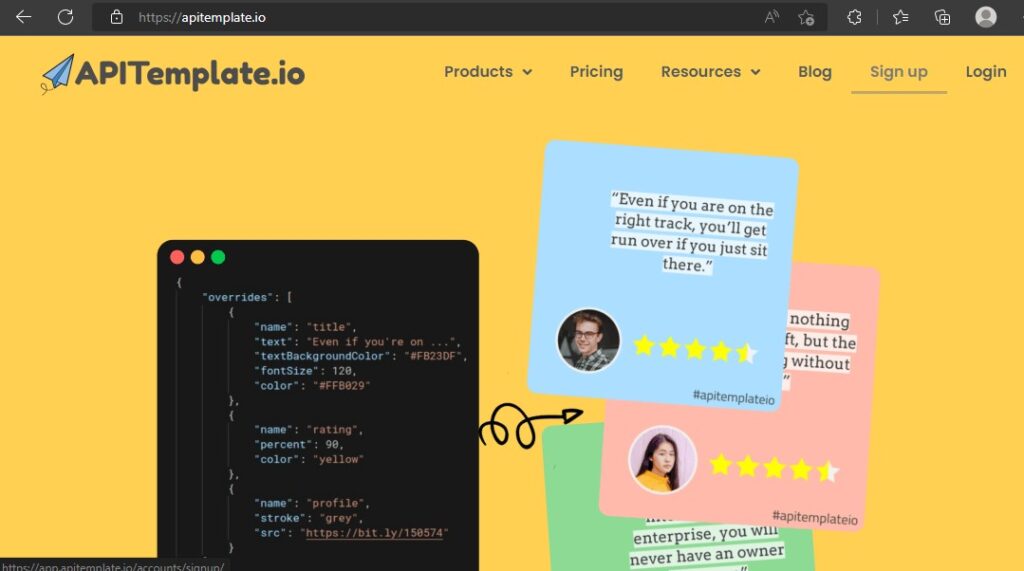
Step 2. Once you have an account, log in and navigate to Manage Templates tab.
Step 3. Click on the New PDF Template button.
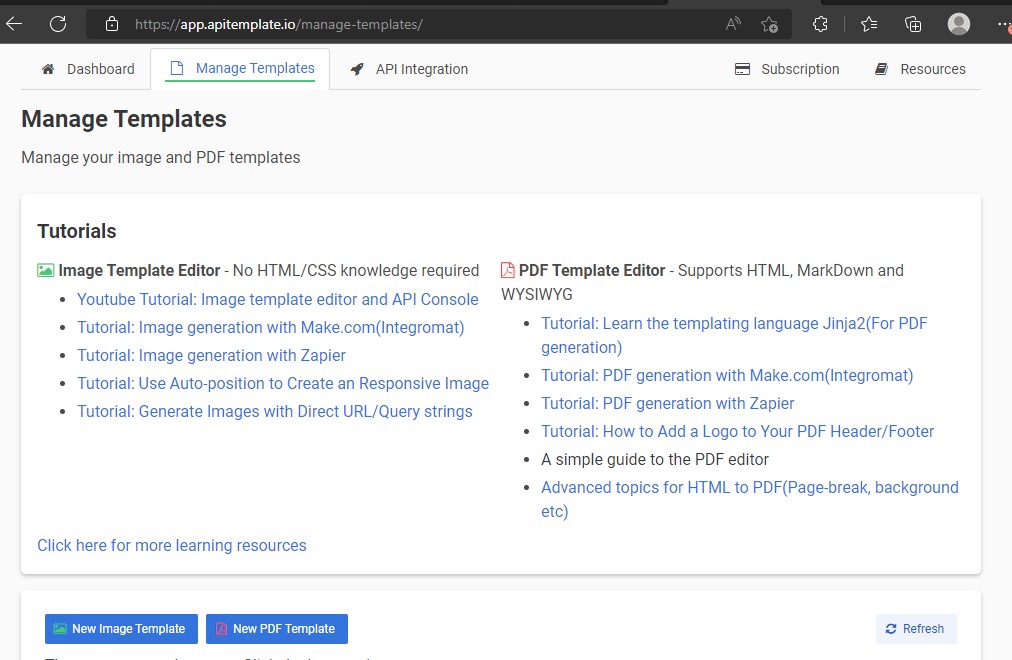
Step 4. Select Sample Invoice Template 1 and click on Create button.
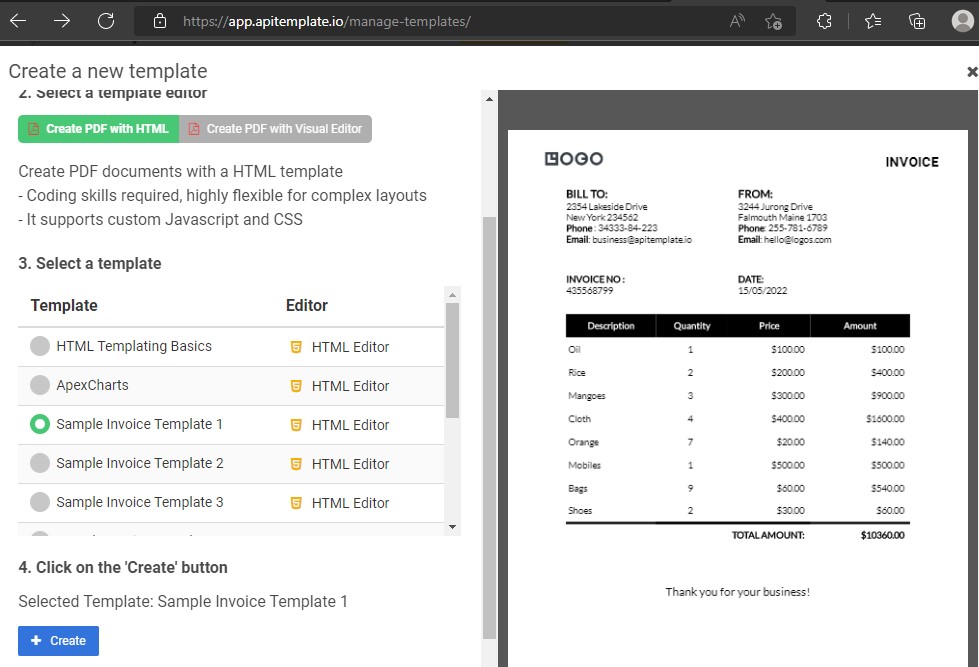
Step 5. Now, navigate to the API Integration tab and copy your API key and save it somewhere safe.
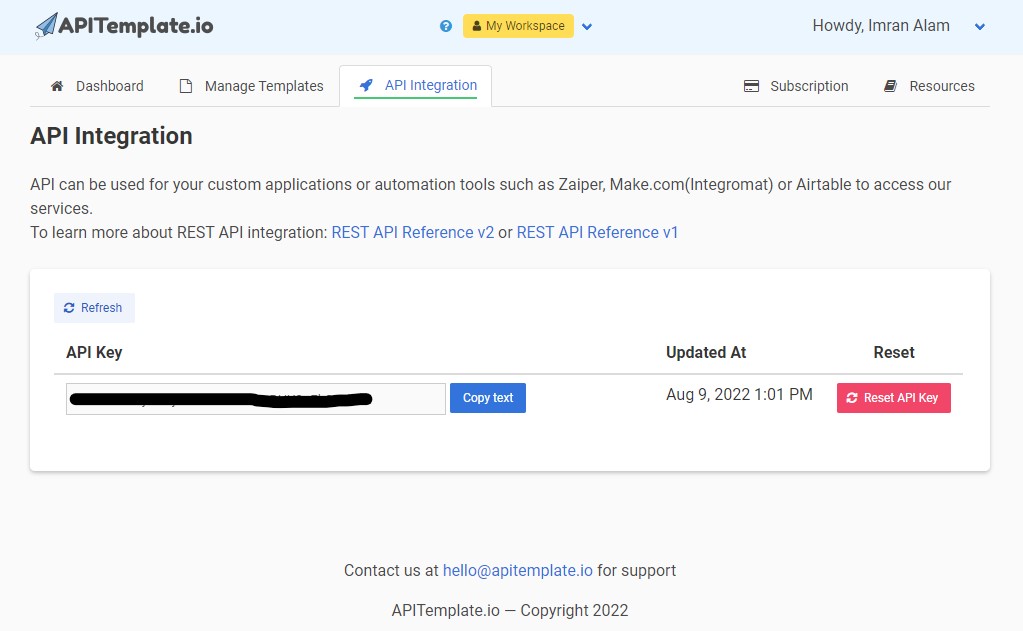
2.3 Generating PDF with C#
To generate a PDF using APITemplate.io, use the below code:
using Newtonsoft.Json;
using System.Text;
var client = new HttpClient();
var url = "https://rest.apitemplate.io/v2/create-pdf?template_id=YOUR_TEMPLATE_ID";
var payload = new
{
date = "15/05/2022",
invoice_no = "435568799",
sender_address1 = "3244 Jurong Drive",
sender_address2 = "Falmouth Maine 1703",
sender_phone = "255-781-6789",
sender_email = "[email protected]",
rece_addess1 = "2354 Lakeside Drive",
rece_addess2 = "New York 234562 ",
rece_phone = "34333-84-223",
rece_email = "[email protected]",
items = new[] {
new {
item_name = "Oil",
unit = 1,
unit_price = 100,
total = 100
},
new {
item_name = "Rice",
unit = 2,
unit_price = 200,
total = 400
},
new {
item_name = "Mangoes",
unit = 3,
unit_price = 300,
total = 900
},
new {
item_name = "Cloth",
unit = 4,
unit_price = 400,
total = 1600
},
new {
item_name = "Orange",
unit = 7,
unit_price = 20,
total = 1400
},
new {
item_name = "Mobiles",
unit = 1,
unit_price = 500,
total = 500
},
new {
item_name = "Bags",
unit = 9,
unit_price = 60,
total = 5400
},
new {
item_name = "Shoes",
unit = 2,
unit_price = 30,
total = 60
}
},
total = "total",
footer_email = "[email protected]"
};
var json = JsonConvert.SerializeObject(payload);
var data = new StringContent(json, Encoding.UTF8, "application/json");
client.DefaultRequestHeaders.Add("X-API-KEY", "YOUR_API_KEY");
var response = await client.PostAsync(url, data);
var responseString = await response.Content.ReadAsStringAsync();
Console.WriteLine(responseString);
In the above code, you will need to replace YOUR_TEMPLATE_ID
and YOUR_API_KEY
with the actual values. You can find your template ID in the APITemplate.io Dashboard, and your API key in the API Integration section.
Once you have made the necessary substitutions, run the code and you should see a JSON response that looks something like this:
{
"download_url":"PDF_URL",
"transaction_ref":"8cd2aced-b2a2-40fb-bd45-392c777d6f6",
"status":"success",
"template_id":"YOUR_TEMPLATE_ID"
}
The download_url field contains a link to your generated PDF. Click on the link to download or view the PDF.
You can also access the PDF via the API by making a GET request to the URL in the download_url
field.
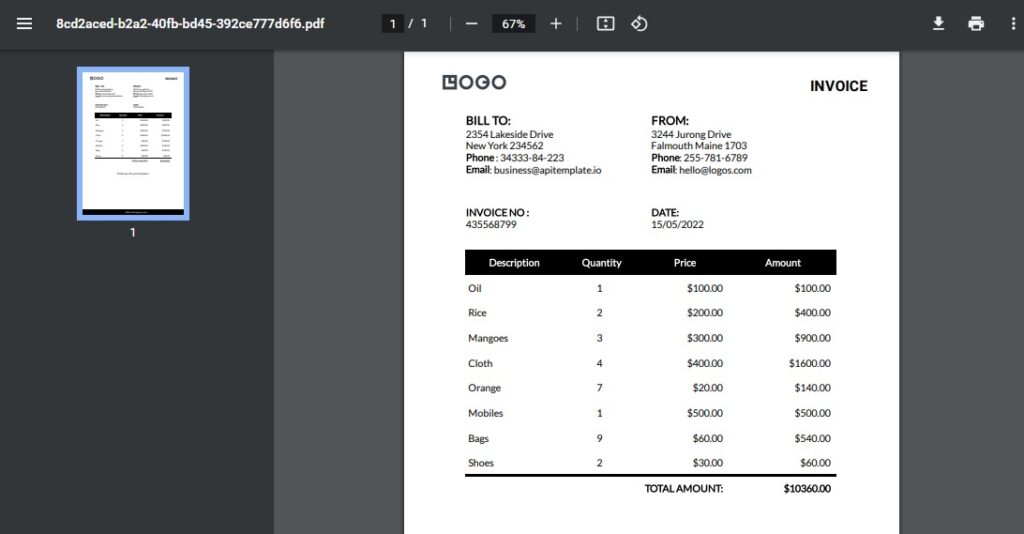
3. Conclusion
In this article, we have shown you how to generate a PDF with Puppeteer Sharp and C#.
In the second part of the article, we have also shown you the benefits and how to easily generate a PDF via the REST API with APITemplate.io.
Sign up for a free account with us now and start automating your PDF generation.