1. Introduction
PHP is one of the most powerful server-side scripting languages available today. This programming language is used by developers to create websites that are dynamic and interactive.
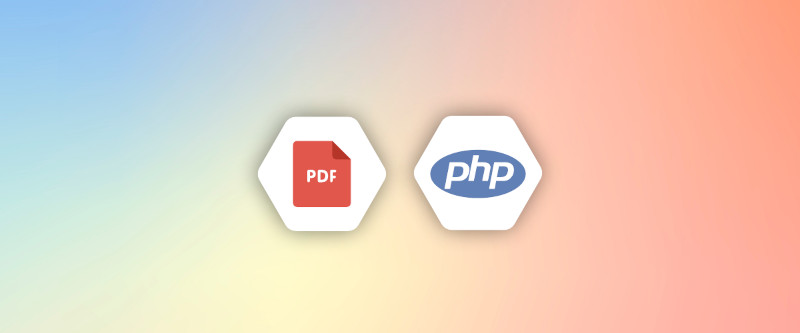
PDF is an abbreviation for Portable Document Format, which captures all the elements in a file and allows people to easily present documents irrespective of the application used. PHP has some powerful libraries that can convert executable codes into PDF documents.
Imagine if you were given a task to include PDF generation in your PHP-based web application, knowing how to use PHP to create PDF documents would come in very handy.
In this article, I’ll demonstrate how to create PDF files with third-party PHP PDF libraries like FPDF, TCPDF, DOMPDF, html2PDF and APITemplate.io’s REST API.
2. PHP Libraries for PDF Generation
PHP provides a range of libraries that can easily assist you in converting your PHP files into PDF documents. I compiled a list of simple libraries, taking implementation, functions, and use cases into account.
2.1 FPDF
FPDF(Free Portable Document Format) is a PHP class that includes numerous functions for creating and editing PDFs for free. The FPDF class supports a variety of features like:
- Image support (PNG, JPEG, and GIF)
- Page headers
- Page format
- Footers
- Automatic page break, and many more
However, because it is a third-party library, it requires some processes before you can use it. You will need to:
- Download the FPDF class for free from the FPDF website.
- Create a folder and extract the FPDF package into it. Let’s say that you have downloaded and extracted the FPDF package inside a folder called fpdf (or any name you like).
- Create a new PHP file called scriptToPDF.php inside the same folder and insert the code snippet below. Save the file and try to access it through your localhost : http://localhost/fpdf/scriptToPDF.php
<?php
require('./fpdf.php');
class myPDF extends FPDF {
// Page header
function Header() {
// Set font family to Arial bold
$this->SetFont('Times','B',14);
// Move to the right
$this->Cell(276,5, 'HOW TO GENERATE PDF USING FPDF');
//Sets the text color
$this->SetTextColor(0,0,255);
//Line break
$this->Ln(20);
// Header
$this->Cell(200,10,'FPDF DOCUMENTATION',0,0,'C');
// Line break
$this->Ln(20);
}
// Page footer
function Footer() {
// Position at 1.5 cm from bottom
$this->SetY(-25);
// Arial italic 8
$this->SetFont('Arial','I',8);
// Page number
$this->Cell(0,10,'Page ' .
$this->PageNo() . '/{nb}',0,0,'C');
}
// header Attributes
function headerAttributes() {
$this->SetFont('Times','B', 10);
$this->Cell(30,10,'Attributes',1,0,'C');
$this->Cell(45,10,'Description',1,0,'C');
$this->Cell(60,10,'How to Use',1,0,'C');
$this->Cell(40,10,'Tutorials',1,0,'C');
$this-the >Ln();
}
}
// Instantiation of FPDF class
$pdf = new myPDF();
// Define an alias for number of pages
$pdf->AliasNbPages();
$pdf->AddPage();
$pdf->headerAttributes();
$pdf->SetFont('Times','',14);
$pdf->Output();
?>
This will create a PDF document with a header, header attributes, and a footer. You can also use FPDF to add a link, draw a line, and perform other functions. More information has been provided in the documentation.
2.2 TCPDF
The TCPDF PHP library makes it simple to generate a PDF document. You can easily accomplish this by cloning the GitHub repository. After cloning the repository, you can import this library into your PHP file. The TCPDF library also supports a variety of functions like:
- Manage page headers and footers automatically
- Text rendering options -Processes for publishing XHTML + CSS code, JavaScript, and Forms.
- Supports JPEG, PNG, and SVG images natively.
- Page breaks, line breaks, and text alignments are all automated.
- Page grouping and automatic page numbering, and many more.
To enable it to work, it requires the following:
- Clone the repository
- Create a file called ‘myPDF.php’ (or any other name you want) inside the folder you cloned and insert the following code snippet.
<?php
// Include the main TCPDF library (search for installation path).
require_once('tcpdf.php');
// create new PDF document
$pdf = new TCPDF(PDF_PAGE_ORIENTATION, PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false);
// set document information
$pdf->SetCreator(PDF_CREATOR);
$pdf->SetAuthor('Nicole Asuni');
$pdf->SetTitle('PDF file using TCPDF');
// set default header data
$pdf->SetHeaderData(PDF_HEADER_LOGO, PDF_HEADER_LOGO_WIDTH, PDF_HEADER_TITLE.' 006', PDF_HEADER_STRING);
// set header and footer fonts
$pdf->setHeaderFont(Array(PDF_FONT_NAME_MAIN, '', PDF_FONT_SIZE_MAIN));
$pdf->setFooterFont(Array(PDF_FONT_NAME_DATA, '', PDF_FONT_SIZE_DATA));
// set default monospaced font
$pdf->SetDefaultMonospacedFont(PDF_FONT_MONOSPACED);
// set margins
$pdf->SetMargins(20, 20, 20);
$pdf->SetHeaderMargin(PDF_MARGIN_HEADER);
$pdf->SetFooterMargin(PDF_MARGIN_FOOTER);
// set auto page breaks
$pdf->SetAutoPageBreak(TRUE, PDF_MARGIN_BOTTOM);
// set image scale factor
$pdf->setImageScale(PDF_IMAGE_SCALE_RATIO);
// add a page
$pdf->AddPage();
$html = <<<EOD
<h1>TCPDF PHP Library is easy!</h1>
<i>This is an example of the TCPDF library.</i>
<p>I am using the default header and footer</p>
<p>Please check the source code documentation for more examples of using TCPDF.</p>
EOD;
// Print text using writeHTMLCell()
$pdf->writeHTMLCell(0, 0, '', '', $html, 0, 1, 0, true, '', true);
// $pdf->writeHTML($html, true, false, true, false, '');
// add a page
$pdf->AddPage();
$html = '<h4>Second page</h4>';
$pdf->writeHTML($html, true, false, true, false, '');
// reset pointer to the last page
$pdf->lastPage();
//Close and output PDF document
$pdf->Output('example.pdf', 'I');
?>
This generates a PDF document using the TCPDF’s library default header and footer. You can find more examples of how to use the library on their website.
2.3 DOMPDF
DomPDF is a PHP-based HTML to PDF converter. It is a library that reads HTML and CSS documents (including inline styles and external stylesheets) and converts them to an automatically generated PDF document. The dompdf library supports many features like:
- CSS 2.1 and a few CSS3 properties, including @import, @media, and @page rules
- The majority of HTML 4.0 presentational attributes
- Compatibility with complex tables
- Support for images (gif, png (8, 24, and 32 bit with alpha channel), bmp, and jpeg).
- No reliance on third-party PDF libraries, among other advantages.
** Note: To enable it to function, PHP version 7.1 or higher is required.
This library can be easily installed via composer:
composer require dompdf/dompdf
Or you can choose to clone the repository on Github to your system. After cloning the repository, create a file called index.php
inside the folder you cloned and insert the following code snippet.
<?php
// Include autoloader
require_once 'dompdf/autoload.inc.php';
// Reference the Dompdf namespace
use Dompdf\Dompdf;
// Instantiate and use the dompdf class
$dompdf = new Dompdf();
$html='
<style>
table {
font-family: arial;
width:400px;
border-collapse: collapse;
}
td, th {
border: 1px solid black;
text-align: left;
padding: 8px;
}
tr:nth-child(even) {
background-color: grey;
}
</style>
<h1>Welcome to api.template</h1>
<h3>List of Registered users </h3>
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>Date of Birth</th>
<th>Country</th>
</tr>
<tr>
<td>Caroline White</td>
<td>50</td>
<td>20-12-1960</td>
<td>Germany</td>
</tr>
<tr>
<td>Jane Dutch</td>
<td>35</td>
<td>20-12-1978</td>
<td>Kenya</td>
</tr>
</table>
';
// Load HTML content
$dompdf->loadHtml($html);
// (Optional) Setup the paper size and orientation
$dompdf->setPaper('A4', 'landscape');
// Render the HTML as PDF
$dompdf->render();
// Output the generated PDF to Browser
$dompdf->stream();
?>
This code generates a PDF document with the following HTML and CSS layout.
2.4 HTML2PDF
The HTML2PDF API makes it simple to convert web pages to PDF. It reads valid HTML codes and converts them to PDF format, allowing it to generate documents such as receipts and manuals, among many others. It should be noted that specific tags must be implemented to use the html2pdf API, therefore, it is recommended that you write your own HTML code. It utilizes TCPDF.
It has a lot of cool features, such as:
- The ability to customize the page size and margins
- Allows you to personalize the layout by changing the fonts and colors to your liking.
- Enables you to customize your headers, footers, and offsets.
- Allows you to add watermarks to your PDF documents.
- Uses encryption or passwords to protect your PDF from unauthorized printing and copying.
To use the HTML2PDF API, you will need:
- PHP version 5.6 or higher
- The mbstring or gd PHP extension installed
The recommended way to install the HTML2PDF is via composer:
composer require spipu/html2pdf
Create a new file in the root folder and paste the following code into it.
<?php
require __DIR__.'\autoload.php';
use Spipu\Html2Pdf\Html2Pdf;
$html2pdf = new Html2Pdf();
$html='
<h1>HTML2PDF is easy</h1>
<p>The HTML2PDF API makes it simple to convert web pages to PDF.</p>
<p>It should be noted that specific tags must be implemented to use the html2pdf</p>
'
$html2pdf->writeHTML($html);
$html2pdf->output();
?>
This code converts the HTML into a PDF document.
2.5 The comparison of HTML2PDF, TCPDF, DomPDF and FPDF
To provide a comparison of popular PHP libraries that handle PDF generation, we’ll look at four widely used ones: HTML2PDF, TCPDF, DomPDF and FPDF. Each library offers different capabilities, focuses, and interfaces, making them suitable for various use cases.
Here’s a detailed table summarizing their features, compatibility, ease of use, and specific functionalities:
Feature | HTML2PDF | TCPDF | DomPDF | FPDF |
---|---|---|---|---|
Based On | TCPDF | Custom PDF engine | Custom PDF engine | Custom PDF engine |
HTML/CSS Support | Full HTML/CSS | Extensive HTML/CSS | Limited HTML/CSS | No HTML/CSS |
PHP Version Compatibility | PHP 5.6 and newer | PHP 5.3 and newer | PHP version 7.1 or higher | At least PHP 5.1 and is compatible with PHP 7 and PHP 8 |
UTF-8 Support | Yes | Yes | Yes | No |
Performance | Moderate | High | Moderate | High |
Ease of Use | Easy | Moderate | Easy | Easy |
Header/Footer Management | Supported | Supported | Supported | Manual |
Image Support | Yes | Yes | Yes | Yes |
JavaScript | No | No | No | No |
Output to file/stream | Yes | Yes | Yes | Yes |
Page Orientation & Size | Customizable | Highly customizable | Customizable | Customizable |
Documentation | Good | Extensive | Adequate | Adequate |
Community Support | Moderate | Strong | Strong | Moderate |
This comparison should help determine which library best fits specific project requirements, whether it’s comprehensive HTML rendering, support for older PHP versions, or advanced PDF customization features.
3. Generating PDF with APITemplate.io using REST API
APITemplate.io is a robust cloud-based API platform that makes it simple to generate REST APIs as PDF documents by using already created templates. It currently supports HTML to PDF conversions and lets you preview your PDF before downloading.
It includes some great features, such as:
- Convert HTML to PDF or using a reusable template
- It is compatible with CSS, JavaScript, PHP, and Python
- APITemplate.io Integrates with Zapier, Integromat, n8n, Airtable, UiPath, and other No-code platforms
- Custom footer and header with page number and total pages are supported.
To use APITemplate.io, you need to do the following:
- Signup at app.apitemplate.io/accounts/signup/ to create an account
- In the
Manage Templates
page, click onCreate PDF Template
button to create a new template - Post and manipulate your JSON data however you like along with the parameters to generate a PDF!
<?php
function generate($template_id,$api_key, $data) {
$url = "https://rest.apitemplate.io/v2/create-pdf?template_id=" . $template_id;
$headers = array("X-API-KEY: ".$api_key);
$curl = curl_init();
if ($data) curl_setopt($curl, CURLOPT_POSTFIELDS, $data);
curl_setopt($curl, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_HTTPHEADER, $headers);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
$result = curl_exec($curl);
curl_close($curl);
if (!$result) {
return null;
}else{
$json_result = json_decode($result, 1);
if($json_result["status"]=="success"){
return $json_result["download_url"];
}else{
return null;
}
}
}
$tempate_id = "79667b2b1876e347";
$api_key = "6fa6g2pdXGIyHRhVlGh7U56Ada1eF";
$json_payload='{ "invoice_number": "INV38379", "date": "2021-09-30", "currency": "USD", "total_amount": 82542.56 }';
echo generate($tempate_id,$api_key,$json_payload);
?>
This endpoint generates a PDF document containing JSON data and the template.
4. Conclusion
In this article, we discussed how to generate PDF files from HTML files using PHP.
We also went over some of the libraries, including htmltopdf, tcpdf, dompdf, fpdf, and APITemplate.io. We also compared them in terms of features, implementation, functions, and use cases.
If I had to suggest a tool, I would recommend APITemplate.io, this is because it can help you generate PDFs quickly and is compatible with CSS, JavaScript, Java, and Python.
Get started with automating your PDF generation by signing up for a free account with us today.