In many real-world applications, PDFs need to be generated from HTML content or directly from a website URL. Previously, it was necessary to write a lot of custom code to do this, which took a lot of time. Now, there are many libraries and tools available that can generate PDFs in just a few lines of code.
In this article, we will explore some approaches for generating PDFs from HTML using PHP, including libraries such as TCPDF, Dompdf, and mPDF.
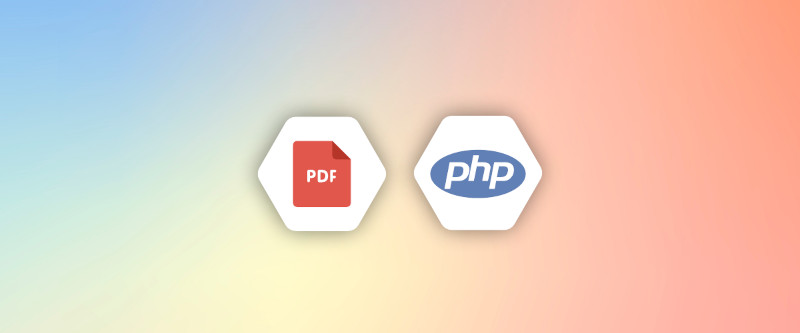
We will also discuss using APITemplate.io, an API-based PDF generation platform, which provides an easy way to generate and manage PDFs using templates.
Converting HTML to PDF using PHP Libraries
i. TCPDF
TCPDF is a PHP library used to generate PDFs from HTML and other types of content. It is highly customizable and offers a wide range of features, including support for different page formats and fonts, barcodes, and encryption. TCPDF is widely used in web applications for generating invoices, reports, and other types of documents.
Generate PDF from a website URL
require_once('tcpdf/tcpdf.php');
// Fetch website content
$websiteContent = file_get_contents('https://google.com');
// Create new PDF document
$pdf = new TCPDF();
// Add a page
$pdf->AddPage();
// Write HTML content
$pdf->writeHTML($websiteContent);
// Output PDF
$pdf->Output('google.pdf', 'I');
In the above code, we are doing the following things:
First, we are fetching the content of the website with
file_get_contents
Then create a new PDF document with TCPDF.
We are adding a page to the PDF document.
The
writeHTML
method is used to write the website content to the PDF document.The
Output
method is used to display the PDF in the browser with the filenamegoogle.pdf
Generate PDF from Custom HTML content
require_once('tcpdf/tcpdf.php');
// Create new PDF document
$pdf = new TCPDF();
// Add a page
$pdf->AddPage();
// Custom HTML content
$html = "<h1>Hello, World!</h1>";
// Write HTML content
$pdf->writeHTML($html);
// Output PDF
$pdf->Output('custom.pdf', 'I');
In the above code, we are doing the following things
- First, we are creating a new PDF document with TCPDF.
- Then add a page to the PDF document.
- Using
writeHTML
method to write the custom HTML content to the PDF document. - And finally, output the pdf to the file path.
ii. Dompdf
Dompdf is a PHP library that can generate PDFs from HTML content. It supports CSS, HTML5, and JavaScript, and can also handle Unicode and complex tables. Dompdf is simple to use and can easily integrate with PHP applications. It is often used for generating invoices, reports, and other types of documents.
Generate PDF from a website URL
require 'vendor/autoload.php';
use Dompdf\Dompdf;
// Fetch website content
$websiteContent = file_get_contents('https://google.com');
$dompdf = new Dompdf();
$dompdf->loadHtml($websiteContent);
$dompdf->render();
$dompdf->stream();
Above code uses the Dompdf library to convert HTML content to PDF.
file_get_contents()
is used to fetch the content of a website and store it in a variable.- We are then creating a new instance of the Dompdf class.
- The website content is loaded using the
loadHtml()
method. - The
render()
method is called to generate the PDF. - The
stream()
method is used to output the PDF to the browser.
Generate PDF from Custom HTML content
require 'vendor/autoload.php';
use Dompdf\Dompdf;
$html = "<h1>Hello, World!</h1>";
$dompdf = new Dompdf();
$dompdf->loadHtml($html);
$dompdf->render();
$dompdf->stream();
In the above PHP code, we are doing the following things
- First, we require the
autoload.php file that
loads the necessary classes and functions for the Dompdf library. - We then create a variable called
$html
which contains the HTML content that we want to convert to PDF. - We are creating a new instance of Dompdf and then loading the content using
loadHtml()
method. - The
render()
method is called to generate the PDF. - The
stream()
method is used to output the PDF to the browser.
iii. mPDF
mPDF is a PHP library that allows for the easy generation of PDF files from HTML content. It supports CSS, HTML5, and JavaScript, and is capable of creating complex documents such as invoices, reports, and catalogs. mPDF is highly customizable and can be used to add images, watermarks, and other features to PDFs.
Generate PDF from a website URL
require_once __DIR__ . '/vendor/autoload.php';
$mpdf = new \Mpdf\Mpdf();
// Fetch website content
$websiteContent = file_get_contents('https://google.com');
$mpdf->WriteHTML($websiteContent);
$mpdf->Output();
The PHP code uses the mPDF library to generate PDFs from website content. The code does the following:
- First we are creating a new instance of the mPDF class.
- Then fetching the content of a website using
file_get_contents()
. - Calls the
WriteHTML()
method to write the website content to the PDF document. - Calls the
Output()
method to output the PDF to the browser.
The resulting PDF will contain the content of the website specified in the file_get_contents()
call.
Generate PDF from Custom HTML content
require_once __DIR__ . '/vendor/autoload.php';
$mpdf = new \Mpdf\Mpdf();
$html = "<h1>Hello, World!</h1>";
$mpdf->WriteHTML($html);
$mpdf->Output();
The above code uses mPDF libraries and generates PDFs.
- First, we are creating a new instance of Mpdf class.
- The HTML content that needs to be converted to PDF is stored in a variable called
$html
. - The
WriteHTML()
method is used to write the content to the PDF document. - The
Output()
method is called to output the PDF to the browser.
Converting HTML to PDF using APITemplate.io
Above are some examples of how we can use libraries in PHP to convert HTML to PDF and web pages to PDF. However, generating PDFs using templates or keeping track of generated PDFs requires additional work.
We need to create our own PDF generator tracker to keep track of the generated files. If we want to use custom templates, such as invoice generators, we also need to create and manage those templates.
APITemplate.io‘s HTML to PDF API is the perfect solution for all of the above use cases. In addition, APITemplate.io uses a chromium-based PDF rendering engine that supports full HTML, content, and CSS.
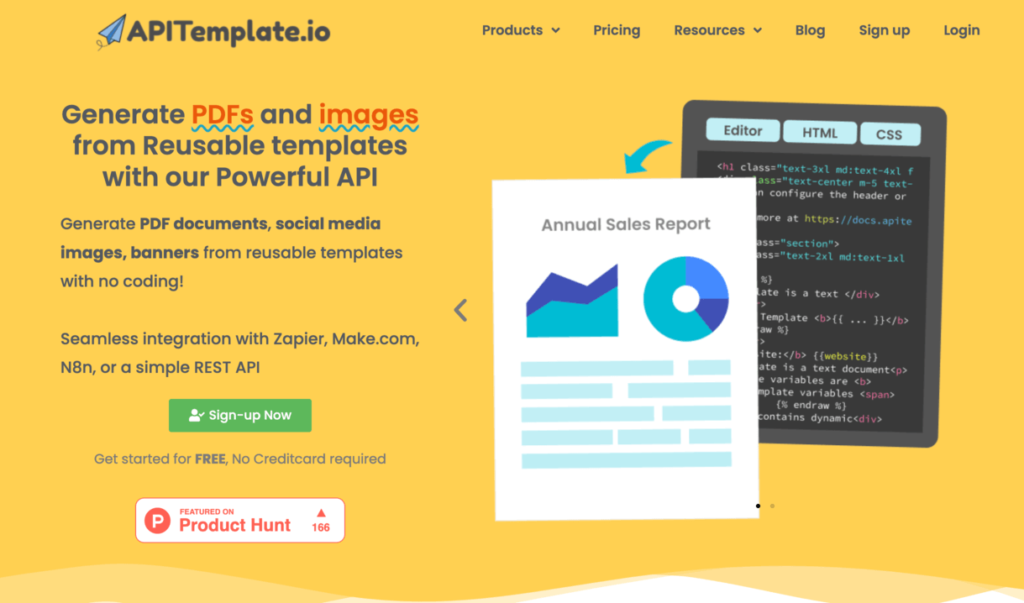
Let’s see how we can use APITemplate.io to generate PDFs.
i. Generate PDF from HTML Template
APITemplate.io allows you to manage your templates. Go to “Manage Templates” from the dashboard.
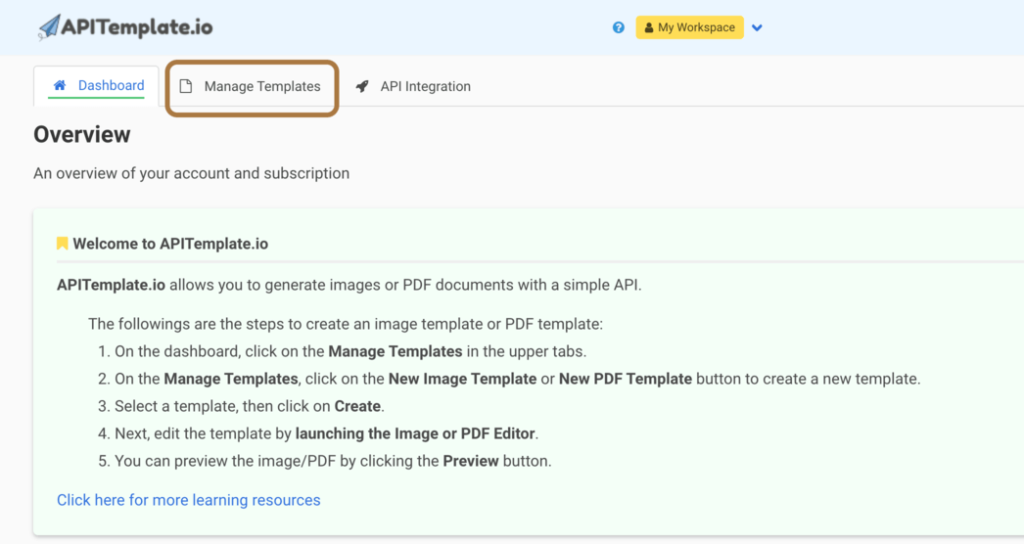
From Manage Template, you can create your own templates. The following is a sample invoice template. There are many templates available that you can choose from and customize based on your requirements.
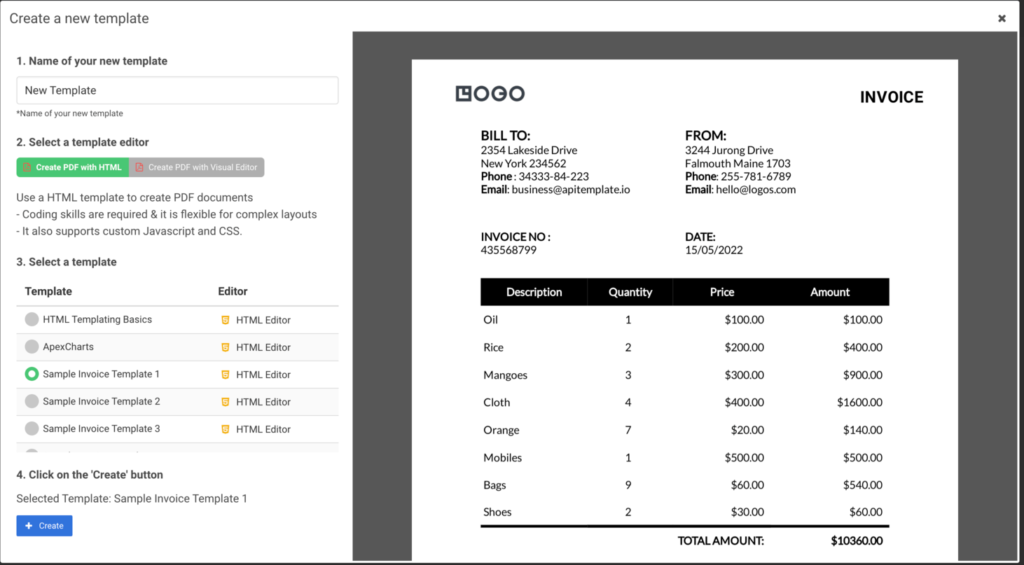
To start using APITemplate.io APIs, you need to obtain your API Key, which can be obtained from the API Integration
tab.
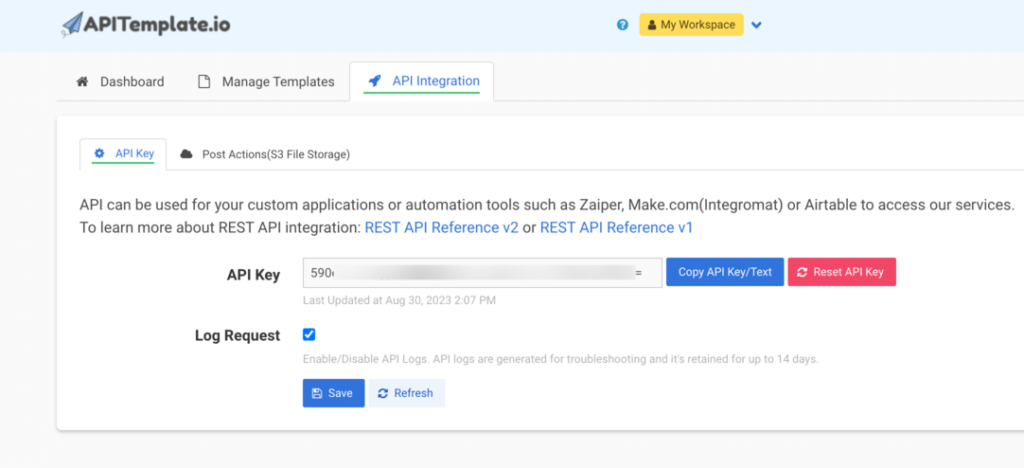
Now that you have your APITemplate account ready, let’s take some action and integrate it with our application. We will use the template to generate PDFs.
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize HTTP client
$client = new Client();
// API URL
$url = "https://rest.apitemplate.io/v2/create-pdf?template_id=YOUR_TEMPLATE_ID";
// Payload data
$payload = [
'date' => "15/05/2022",
'invoice_no' => "435568799",
'sender_address1' => "3244 Jurong Drive",
'sender_address2' => "Falmouth Maine 1703",
'sender_phone' => "255-781-6789",
'sender_email' => "[email protected]",
'rece_addess1' => "2354 Lakeside Drive",
'rece_addess2' => "New York 234562",
'rece_phone' => "34333-84-223",
'rece_email' => "[email protected]",
'items' => [
['item_name' => "Oil", 'unit' => 1, 'unit_price' => 100, 'total' => 100],
['item_name' => "Rice", 'unit' => 2, 'unit_price' => 200, 'total' => 400],
['item_name' => "Mangoes", 'unit' => 3, 'unit_price' => 300, 'total' => 900],
['item_name' => "Cloth", 'unit' => 4, 'unit_price' => 400, 'total' => 1600],
['item_name' => "Orange", 'unit' => 7, 'unit_price' => 20, 'total' => 1400],
['item_name' => "Mobiles", 'unit' => 1, 'unit_price' => 500, 'total' => 500],
['item_name' => "Bags", 'unit' => 9, 'unit_price' => 60, 'total' => 5400],
['item_name' => "Shoes", 'unit' => 2, 'unit_price' => 30, 'total' => 60],
],
'total' => "total",
'footer_email' => "[email protected]",
];
// Set headers
$headers = [
'X-API-KEY' => 'YOUR_API_KEY',
'Content-Type' => 'application/json',
];
try {
// Make the POST request
$response = $client->post($url, [
'json' => $payload,
'headers' => $headers,
]);
// Read the response
$responseString = json_encode(json_decode($response->getBody()));
// Print the response
echo $responseString;
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
And if we check the response_string
, we have the following:
{
"download_url":"PDF_URL",
"transaction_ref":"8cd2aced-b2a2-40fb-bd45-392c777d6f6",
"status":"success",
"template_id":"YOUR_TEMPLATE_ID"
}
In the above code, it’s very easy to use ApiTemplate to convert HTML to PDF because we don’t need to install any other library. We just need to call one simple API and use our data as a request body, and that’s it!
You can use the download_url
from the response to download or distribute the generated PDF.
ii. Generate PDF from website URL
ApiTemplate also supports generating PDFs from website URLs.
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize HTTP client
$client = new Client();
// API key and template ID
$api_key = "YOUR_API_KEY";
$template_id = "YOUR_TEMPLATE_ID";
// Data payload
$data = [
'url' => "https://en.wikipedia.org/wiki/Sceloporus_malachiticus",
'settings' => [
'paper_size' => "A4",
'orientation' => "1",
'header_font_size' => "9px",
'margin_top' => "40",
'margin_right' => "10",
'margin_bottom' => "40",
'margin_left' => "10",
'print_background' => "1",
'displayHeaderFooter' => true,
'custom_header' => '<style>#header, #footer { padding: 0 !important; }</style>
<table style="width: 100%; padding: 0px 5px;margin: 0px!important;font-size: 15px">
<tr>
<td style="text-align:left; width:30%!important;"><span class="date"></span></td>
<td style="text-align:center; width:30%!important;"><span class="pageNumber"></span></td>
<td style="text-align:right; width:30%!important;"><span class="totalPages"></span></td>
</tr>
</table>',
'custom_footer' => '<style>#header, #footer { padding: 0 !important; }</style>
<table style="width: 100%; padding: 0px 5px;margin: 0px!important;font-size: 15px">
<tr>
<td style="text-align:left; width:30%!important;"><span class="date"></span></td>
<td style="text-align:center; width:30%!important;"><span class="pageNumber"></span></td>
<td style="text-align:right; width:30%!important;"><span class="totalPages"></span></td>
</tr>
</table>'
]
];
try {
// Make the POST request
$response = $client->post(
"https://rest.apitemplate.io/v2/create-pdf-from-url",
[
'json' => $data,
'headers' => [
'X-API-KEY' => $api_key
]
]
);
// Read the response
$responseString = json_encode(json_decode($response->getBody()));
// Print the response
echo "PDF generated successfully: " . $responseString;
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
In the above code, we can provide the URL in the request body along with the settings for the PDF. APITemplate will use this request body to generate a PDF and return a download URL for your PDF.
iii. Generate PDF from custom HTML content
If you want to generate PDFs using your own custom HTML content, ApiTemplate also supports that.
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize HTTP client
$client = new Client();
// API key and template ID
$api_key = "YOUR_API_KEY";
$template_id = "YOUR_TEMPLATE_ID";
// Data payload
$data = [
'body' => "<h1> hello world {{name}} </h1>",
'css' => "<style>.bg{background: red};</style>",
'data' => [
'name' => "This is a title"
],
'settings' => [
'paper_size' => "A4",
'orientation' => "1",
'header_font_size' => "9px",
'margin_top' => "40",
'margin_right' => "10",
'margin_bottom' => "40",
'margin_left' => "10",
'print_background' => "1",
'displayHeaderFooter' => true,
'custom_header' => '<style>#header, #footer { padding: 0 !important; }</style>
<table style="width: 100%; padding: 0px 5px;margin: 0px!important;font-size: 15px">
<tr>
<td style="text-align:left; width:30%!important;"><span class="date"></span></td>
<td style="text-align:center; width:30%!important;"><span class="pageNumber"></span></td>
<td style="text-align:right; width:30%!important;"><span class="totalPages"></span></td>
</tr>
</table>',
'custom_footer' => '<style>#header, #footer { padding: 0 !important; }</style>
<table style="width: 100%; padding: 0px 5px;margin: 0px!important;font-size: 15px">
<tr>
<td style="text-align:left; width:30%!important;"><span class="date"></span></td>
<td style="text-align:center; width:30%!important;"><span class="pageNumber"></span></td>
<td style="text-align:right; width:30%!important;"><span class="totalPages"></span></td>
</tr>
</table>'
]
];
try {
// Make the POST request
$response = $client->post(
"https://rest.apitemplate.io/v2/create-pdf-from-html",
[
'json' => $data,
'headers' => [
'X-API-KEY' => $api_key
]
]
);
// Read the response
$responseString = json_encode(json_decode($response->getBody()));
// Print the response
echo "PDF generated successfully: " . $responseString;
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Similar to generating a PDF from a website URL, the API request above takes the body and CSS as part of the payload to generate a PDF.
Performance Considerations
Generating PDFs from HTML content or website URLs is a common requirement in many real-world applications. While open-source third-party libraries work fine in most cases, they may not be suitable for scaling and handling all edge cases.
This is where APITemplate.io comes in. it’s PDF generation API offers an easy way to generate and manage PDFs using templates. APITemplate.io handles all scaling and edge cases for you, so you don’t need to worry about performance or error situations.
It’s a great solution for generating PDFs at scale without having to worry about the underlying infrastructure.
Conclusion
PDF generation features are now a part of every business application.
We have seen how we can use third-party libraries to generate PDFs if our use case is simple. However, if we have complex use cases, such as maintaining templates, APITemplate.io‘s PDF generator API provides a solution just for that using simple API calls.
Sign up for a free account with us now and start automating your PDF generation.
Libraries: