Introduction
E-commerce has become more popular over the past two years and has become an essential source of income for many companies. One of the most popular needs is the ability to print online transcripts or invoices through your web application, which contains dynamic data based on user activity. Another use case is the need to print website content, whether you have an educational website or a blog. Sometimes the user needs to keep a hard copy of your content, highlighting the need to convert the HTML pages into PDF format.
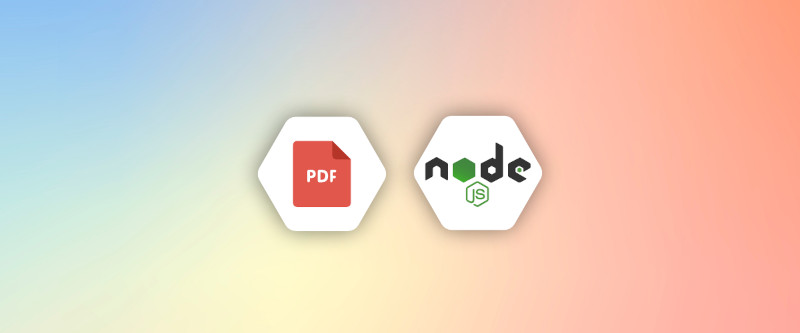
As your data is dynamic in most cases, as the invoice example we introduced earlier, the most suitable way to handle the data and the conversion is to use JavaScript as it provides you with the ability to deal with the data dynamically.
With this need in mind, This article will explore different JavaScript libraries for converting HTML to PDF online and discuss the advantages and disadvantages of each library to help you choose the suitable tool for your application.
Libraries
Suppose we have an invoice that we want our customer to be able to print. Furthermore, we want this invoice to be well-formatted to be printed. There are many libraries and tools available we can use to convert this invoice from HTML format to PDF, and Here we will explore some of the most popular libraries among them.
jsPDF
We can start by introducing jsPDF, jsPDF is an open-source library for generating pdf using only JavaScript. It simply creates a pdf page and applies your formatting to the page.
An example of using the jsPDF with JavaScript:
<!DOCTYPE html>
<html>
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jspdf/1.0.272/jspdf.debug.js"></script>
<script>
function generatePDF() {
var pdf = new jsPDF({
orientation: 'p',
unit: 'mm',
format: 'a5',
putOnlyUsedFonts:true
});
pdf.text("Generate a PDF with JavaScript", 20, 20);
pdf.text("published on APITemplate.io", 20, 30);
pdf.addPage();
pdf.text(20, 20, 'The second page');
pdf.save('jsPDF_2Pages.pdf');
}
</script>
</head>
<body>
<button onclick="generatePDF()">Generate PDF using jsPDF</button>
</body>
</html>
Note that we can change the presentation of the data inside the downloaded PDF file by editing the orientation and format.
We can try the code by simply adding it to a file with HTML format and running it in your browser. You can find more information here.
jsPDF is often combined with Html2Canvas, Html2Canvas is a JavaScript library that can be used to convert your web page into an image, usually, jsPDF and Html2Canvas are used together to take a screenshot of your site and save it in a pdf format.
html2pdf
html2pdf combines jsPDF and Html2Canvas in one library to convert webpages into a PDF.
To be able to convert your webpage to a pdf, we need to:
- import the JavaScript library either by installing it locally in your server using NPM or by including it in your HTML code like the following example.
- Add generatePDF function which will convert the passed section of your webpage into a pdf by calling document.getElementById().
- Add format options in ”opt” variable to be passed to the html2pdf.
- add a button in your HTML page to trigger the generatePDF function.
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2pdf.js/0.10.1/html2pdf.bundle.min.js" integrity="sha512-GsLlZN/3F2ErC5ifS5QtgpiJtWd43JWSuIgh7mbzZ8zBps+dvLusV+eNQATqgA/HdeKFVgA5v3S/cIrLF7QnIg==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script>
function generatePDF() {
const element = document.getElementById('container_content');
var opt = {
margin: 1,
filename: 'html2pdf_example.pdf',
image: { type: 'jpeg', quality: 0.98 },
html2canvas: { scale: 2 },
jsPDF: { unit: 'in', format: 'letter', orientation: 'portrait' }
};
// Choose the element that our invoice is rendered in.
html2pdf().set(opt).from(element).save();
}
</script>
</head>
.
.
.
you can find more information here
Puppeteer
In contrast with all the other libraries, the Puppeteer is not meant to be a library to convert the HTML files into pdf. Instead, it is a tool that automates and controls your browser, which means that we can automate anything that we can do manually on your browser with this tool.
To enable your users to download and export web pages as PDF. First, we should have a Node.js server to add Puppeteer as a dependency for our project.
Second, create a function for generating PDF like Puppeteer_GeneratePDF
where you can add a new browser page using browser.newPage( )
and which section you need to be exported or the page address you need to export.
Then export it using newpage.pdf()
. An example is shown below.
const puppeteer = require('puppeteer');
async function Puppeteer_GeneratePDF(){
// launching browser session
const browser = await puppeteer.launch()
// creating a new page
const newpage = await browser.newPage()
// defining the content to be converted to pdf
const pdfContent = `<!DOCTYPE html><br><html><br>
<title>Example of HTML to PDF using Puppetter</title><br>
<body><br><br>
<h1> Write here your heading</h1><br>
<p>Write here your paragraph.</p><br><br>
</body><br>
</html>
await newpage.setContent(pdfContent)
// exporting generating the pdf from content
await newpage.pdf({ path: 'generated_File_name.pdf', format: 'A4' })
// closing browser session
await browser.close()
}
You can read more about Puppeteer here.
pdf-lib
PDF-lib is another library for generating PDF from HTML, while other libraries support only generating pdf. This library also enables you to edit any existing document and works in Javascript. However, more complexity is introduced with more features added to the library.
An example of code to generate PDF with JavaScript:
<html>
<head>
<meta charset="utf-8" />
<script src="https://unpkg.com/pdf-lib"></script>
</head>
<body>
<iframe id="pdf" style="width: 100%; height: 100%;"></iframe>
</body>
<script>
createPdf();
async function createPdf() {
const pdfDoc = await PDFLib.PDFDocument.create();
const page = pdfDoc.addPage([400, 500]);
page.moveTo(100, 250);
page.drawText('Example Generating PDF with PDF-lib', {
size: 12});
const pdfDataUri = await pdfDoc.saveAsBase64({ dataUri: true });
document.getElementById('pdf').src = pdfDataUri;
}
</script>
</html>
you can read more about pdf-lib from here
Which one is suitable for me?
Answering this question depends on our needs. Suppose we need to have a tool that runs only on the client-side and doesn’t need to allocate resources on the server-side. In that case, the Puppeteer is not a suitable tool for you. Puppeteer is a Node library that only enables the user to control headless Chrome using APIs. All the other libraries work well on the client-side.
On the complexity scale, the jsPDF library is too complex to master because all the elements must be manually encoded, followed by pdf-lib. In comparison, Puppeteer is the least complex tool to automate any browser task, not just converting the webpage into PDF.
When comparing the generated files, we will find that the Puppeteer generates high-resolution files with small size and selectable text while jsPDF and html2pdf generate bigger file sizes with low resolution, and you can’t select the text on them. The reason is that html2pdf and jsPDF take a screenshot of the website content, which cause the text to appear blurry in some cases.
A method to eliminate this blurriness is to configure the html2pdf to use PNG instead of JPEG format, but this will increase the generated PDF file size significantly or use larger resolution when configuring html2pdf.
One of the most important features when selecting the tool/library is its compatibility with browsers. Another disadvantage for Puppeteer will shine as the tool only supports Chrome, and all other browsers are still a work in progress. Finally, Regarding library features, we will find that pdf-lib supports more features than the other libraries, like creating and editing documents and forms.
Conclusion
Throughout this article, We discussed various ways to export your web page into pdf. We had a brief introduction to some of the tools/ libraries like jsPDF, html2pdf, puppeteer and pdf-lib. We also compared them in different properties like complexity, size of generated files, resolution and Features.
Finally, if you want to have a tool with all the features of these libraries and more, in that case, I recommend that you check out APITemplate.io, which is a tool that can help you generate PDF quickly with PDF generating API over the cloud and totally compatible with CSS and JavaScript. It also comes with predefined templates which you can reuse and edit.