1. Introduction
We have often come across different document formats and may need to convert these documents into our suitable choice depending on the context or requirement of a project or task. You may ask, why are document formats important? Aside from maintaining consistency, there can be other reasons like having access to these documents offline.
Our focus here is on HTML to PDF conversion. It is no news that HTML is fantastic for creating interactive web pages, but when it comes to professional documents, not so much. This is where PDF documents come in handy.
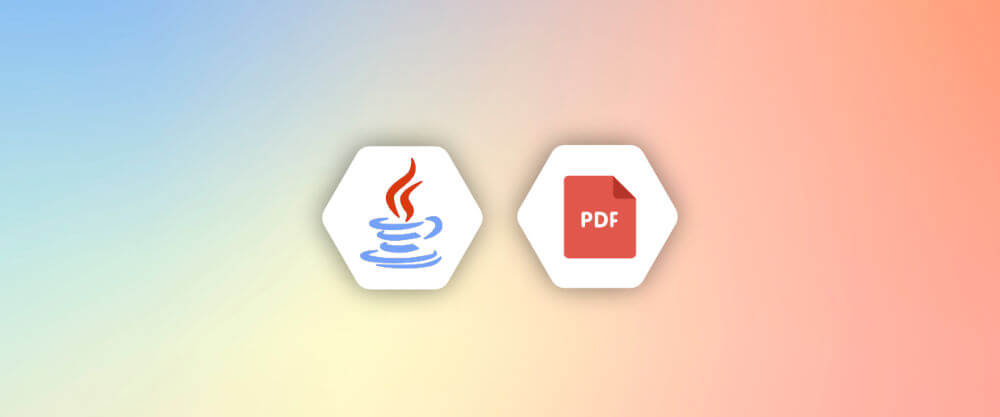
PDF which is an acronym for Portable Document Format are a type of file format that are used for presenting documents. PDFs help preserve the original layout of the document, including the text, fonts, images, and all of those good stuff. Even better, they ensure the document looks exactly as intended when printed.
Maintaining consistency isn’t the only reason why PDFs are great. PDF files can be accessed offline and are more polished than basic HTML pages. They also have better security options and can be easily shared via email, cloud, and other digital means.
OpenPDF is a framework that is used for converting HTML to PDF documents. It uses Java programming language for its conversion.
In this article, we will look at how to convert HTML documents to PDF documents using OpenPDF and Java. Let’s get started!
2. Understanding OpenPDF
OpenPDF is an open-source library (free to use) that provides a framework for creating PDF documents. It is built on the Java programming language and offers developers a powerful tool for converting HTML documents to PDF documents.
Being a Java based framework, it can run on any platform that supports Java and can also seamlessly integrate with Java applications.
As an open-source project, OpenPDF benefits from an active community of developers who contribute to its improvement and provide support. Developers can modify the source code to meet specific needs and ensure the library can be tailored to various use cases.
Why choose OpenPDF for HTML to PDF conversion?
There are several reasons why OpenPDF is a great choice for HTML to PDF conversion. Let’s take a look at some of them:
- Open Source: OpenPDF is free to use and provides a PDF manipulation capability with no-cost.
- Customizable: Developers can modify the source code according to their preferences.
- Easy integration with Java-based applications: OpenPDF can easily integrate with Java-based applications. This makes it suitable for developers in the Java ecosystem.
- Enhanced user experience: OpenPDF allows for the inclusion of interactive elements like bookmarks and hyperlinks.
- Security features: OpenPDF includes support for PDF encryption and digital signatures, helping to secure documents and verify authenticity.
3. Setting Up Your OpenPDF Environment
Getting started with OpenPDF for HTML to PDF conversion involves a few essential steps to set up your development environment. Here’s a step-by-step guide to help you get started:
Step 1: Install Java Development Kit (JDK)
OpenPDF is a Java library, so having the Java Development Kit (JDK) installed on your system is essential. Here’s how to set it up:
- Download the JDK from the official Oracle website or AdoptOpenJDK.
- Follow the installation instructions for your operating system.
Step 2: Set Up Your Development Environment
Download and install your preferred Integration Development Environment (IDE). This can be IntelliJ IDEA, Eclipse, or NetBeans. I will be using NetBeans for this article.
The next step is proceeding to create a New Java Project. You need to open your IDE (Netbeans), and select File > New Project, then, navigate to your project’s directory.
Step 3: Add OpenPDF to Your Project
You need to add the OpenPDF library to your project. This can be done using Maven or Gradle. For this article, I will be using Maven. Here’s how to set up your project using maven:
Using Maven: To create a Maven Project in Netbeans, select File > New Project > Java with Maven + Java Application.
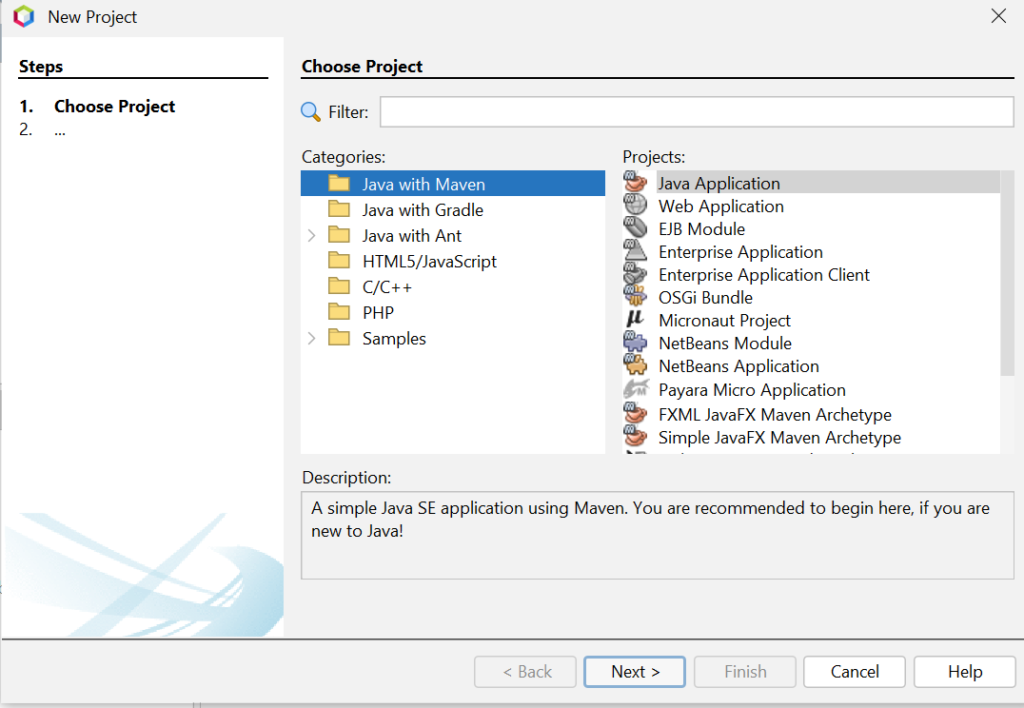
After creating your project, Go to Project Files > pom.xml and add the following OpenPDF dependency to your pom.xml file:
<dependency>
<groupId>com.github.librepdf</groupId>
<artifactId>openpdf</artifactId>
<version>2.0.2</version>
</dependency>
Build your project to ensure the dependency gets downloaded. You should be able to see OpenPDF listed as a dependency. Pretty easy, right?
4. Basic HTML to PDF Conversion
In this section, we will be using OpenPDF to convert a basic HTML code into a PDF document. Firstly, we need to go to our Java file which is located under Source Packages. In the main class, copy and paste the following code snippet:
package com.mycompany.htmltopdf;
import com.lowagie.text.Document;
import com.lowagie.text.Element;
import com.lowagie.text.Paragraph;
import com.lowagie.text.pdf.PdfWriter;
import java.io.FileOutputStream;
public class HtmlToPdf {
public static void main(String[] args) {
String htmlContent = "<html><body><h1>Hello, OpenPDF!</h1><p>This is a sample HTML content converted to PDF.</p></body></html>";
try {
// Print the current working directory
System.out.println("Current working directory: " + System.getProperty("user.dir"));
// Initialize the document and writer
Document document = new Document();
PdfWriter.getInstance(document, new FileOutputStream("output.pdf"));
document.open();
// Create paragraphs manually based on HTML structure
Paragraph h1 = new Paragraph("Hello, OpenPDF!");
h1.setAlignment(Element.ALIGN_CENTER);
h1.setSpacingAfter(10f);
document.add(h1);
Paragraph p = new Paragraph("This is a sample HTML content converted to PDF.");
p.setAlignment(Element.ALIGN_JUSTIFIED);
p.setSpacingAfter(20f);
document.add(p);
// Close the document
document.close();
System.out.println("PDF created successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Remember to use the appropriate package name and class name for your project, build and run the application.
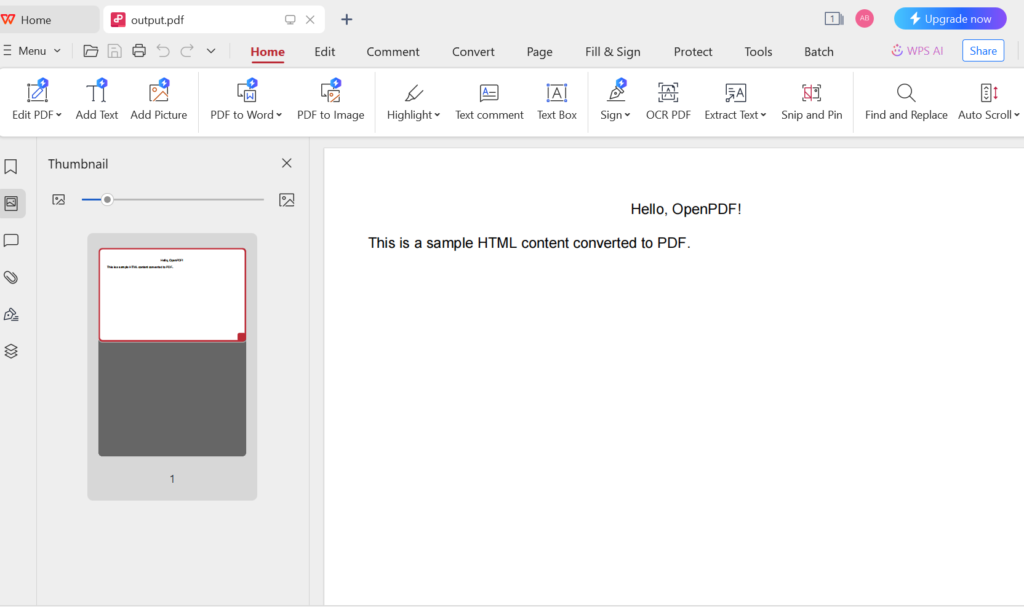
First, we define our class HtmlToPdf within the package com.mycompany.htmltopdf. This is the entry point of our application. We import the necessary classes from the OpenPDF library to get started, including Document, Element, Paragraph, and PdfWriter. Additionally, we imported FileOutputStream from the standard Java library to handle file output operations.
Within the HtmlToPdf class, the main method is defined, which is where our program starts execution. We define a string that contains our HTML content. In this example, it includes a header (<h1>) and a paragraph (<p>).
We then use a try-catch block to handle any potential exceptions during PDF creation. We print the current working directory to the console inside the try block. This helps us verify where the output PDF file will be saved.
Next, we initialize a Document instance, which represents the PDF document. We then create a PdfWriter instance, which writes the content to a file named output.pdf, and open the document to start adding content.
Finally, we close the document, which finalizes the PDF file. A success message “PDF created successfully.” is printed to the console along with the file location.
5. Advanced Features and Customizations in OpenPDF
With OpenPDF, we can customize our PDF documents using advanced features. Here are some key enhancements to look out for:
- Using CSS for Advanced Styling in PDFs: Inline CSS can be applied directly within the HTML tags, whereas external CSS involves linking an external stylesheet. Depending on your preference, you can take advantage of any to style your HTML content.
- Including Headers and Footers: Adding consistent headers and footers across all pages of the PDF enhances readability and professionalism. Headers can include titles, logos, or other information, while footers can have page numbers or document metadata.
- Page Numbering, Embedding Fonts and Images: Adding page numbers to each page helps navigate large documents. Also, custom fonts and images can be embedded in the PDF to maintain a consistent look and feel as per branding requirements.
- Tables and Layouts: Creating tables and managing complex layouts is crucial for businesses. It is used for documents like reports, invoices and catalogs. This makes your documents look more organized.
6. Practical Applications of OpenPDF
Converting HTML to PDF has become unavoidable, especially in the business world. Here are some key reasons why this conversion is important:
- Simplified invoicing: Simplify invoicing processes by converting HTML templates into PDF invoices ensures a professional presentation and increases client satisfaction.
- Efficient Report Generation: Converting HTML reports into PDFs helps with easy distribution and professional printing, which is ideal for sharing insights effectively.
- Safeguard important documents: Converting HTML to PDF helps safeguard important documents for long-term storage. This preserves formatting and content integrity over time, crucial for compliance and record-keeping.
- Legal Clarity: Converting legal documents such as contracts and agreements from HTML to PDF ensures authenticity and tamper-proof integrity.
- Educational Resources: Converting course materials and study guides from HTML to PDF aids easy distribution to students. This simplifies access to these documents and ensures consistent formatting across various devices.
- Manuals and Guides: You can create user manuals, installation guides, and instructional materials in PDF from HTML. This ensures your documents look consistent and professional.
- Marketing Materials: Converting HTML documents of brochures, flyers, and other promotional content into PDFs makes these materials easy to share and look great when printed.
7. Introducing cloud-based API for PDF generation
APITemplate.io is a cloud based solution that offers robust PDF Generation API with full support for HTML to PDF conversion. With its ability to handle HTML, CSS, JavaScript, PHP, Java, and more.
It gives you the power to convert static pages into dynamic contents. It also allows you embed charts, create responsive layouts, use custom fonts, etc, to ensure your PDFs look exactly how you envision them.
Why APITemplate.io is Your Go-To for PDF Generation
To ensure fast, reliable service no matter where your business operates, APITemplate.io supports regional endpoints across the globe. With servers in the US, Singapore, Europe (Germany), and Australia, you can generate PDFs quickly and efficiently.
APITemplate.io minimizes latency and ensures a smooth user experience. This global presence is also particularly beneficial for multinational companies that need consistent performance in different regions.
With our PDF generation API, the possibilities are vast. Here are some of the ways our services support PDF generation:
Method 1: Template-based PDF generation
APITemplate.io allows you to generate PDF documents using our pre-defined templates. These templates can be customized to suit your needs, and also helps you eliminate the stress of manually creating a template from scratch. All you need to do is create an account with us (no credit-card required). This gives you access to all our pre-defined templates and our API key. This API key helps us easily integrate the PDF into our applications. For more information on how to use our template-based PDF generation, check out our existing article on How to convert HTML to PDF using Java.
Method 2: Generate PDFs directly from a website URL
APITemplate.io gives us the ability to generate PDF documents directly from website URLs. All we need to do is provide the URL and this will return a downloadable URL for the PDF. This feature is great when you need to convert an online resource into a PDF. For more information on how to generate PDFs directly from website URLs, check out our existing article on How to convert HTML to PDF using Java.
Method 3: Generate PDFs using custom HTML content
If you already have an existing HTML content you’d like to convert into a PDF document, then this solution is for you. APITemplate.io offers a great way to do this. It takes in your HTML content as the payload data and uses this data to generate your PDF. Check out our existing guide for more information on how to generate PDFs using html content.
8. Conclusion
Don’t just settle for the basics—experiment with OpenPDF and explore its customization options to create interactive documents.
OpenPDF is your go-to solution for converting HTML to PDF in Java. Whether you’re generating invoices, reports, or marketing materials, it ensures your documents are beautifully formatted and easy to distribute.
If you are also exploring ways to take your document generation to the next level- think APITemplate.io.
Sign up now to experience ease when converting your HTML documents to PDFs. Do not forget to take advantage of our PDF services mentioned above. Thanks for reading!