1. Introduction
In the eCommerce world, every order triggers a flurry of documents – order receipts, invoices, shipping labels, packing slips, and more. These documents are often generated as HTML on the fly (e.g. an HTML invoice template filled with order data), but delivering them as PDF is crucial.
PDFs provide a consistent, printer-friendly format that customers and businesses can easily download or print. Unlike raw HTML (which might display differently across browsers or devices), a PDF preserves the exact layout, branding, and formatting as intended.
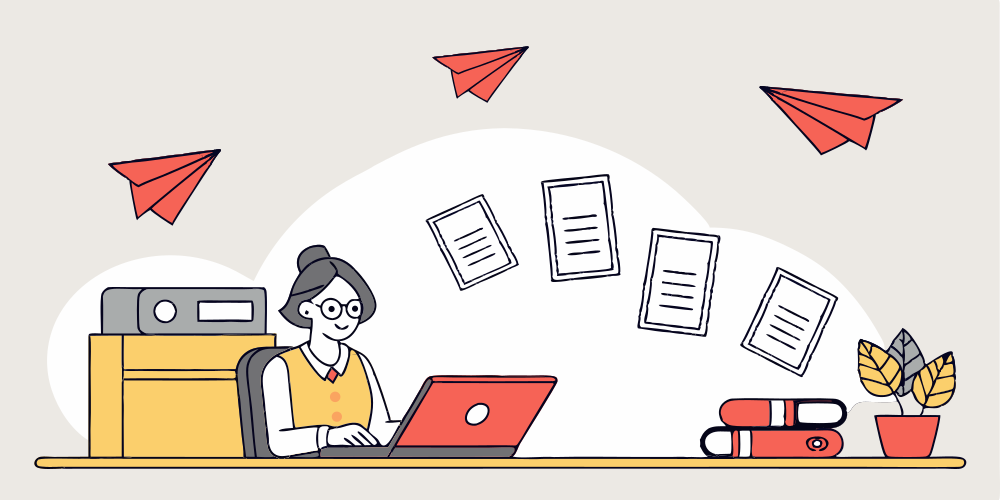
This means an invoice or receipt in PDF will look the same to everyone, reinforcing professionalism and ensuring no information is misaligned.
In this guide you’ll learn why HTML-to-PDF conversion is critical, explore popular open-source options, and see how a cloud API such as APITemplate.io can remove the heavy lifting while keeping full design control.
Key Takeaways
- PDFs are the gold standard for receipts, invoices and shipping labels and customers can print or store them without layout surprises.
- Open-source tools like Puppeteer and wkhtmltopdf work, but they demand server resources, scaling logic and constant maintenance.
- API-based services: especially APITemplate.io: remove the DevOps burden while giving you modern HTML / CSS support, template management and global scalability out of the box.
- A label, invoice or receipt is just one POST request away: design once, send JSON data and receive a download-ready PDF.
- Step-by-step label tutorial and full v2 OpenAPI spec are included below, so you can integrate in minutes.
2. Manual Conversion with Open-Source Tools
There are a few common ways developers traditionally handle HTML to PDF conversion in their applications.
Two popular approaches are using open-source libraries or tools like Puppeteer and wkhtmltopdf. These let you convert HTML content to PDFs within your own environment, without an external service.
Let’s look at how each works:
Puppeteer (Headless Chrome)
How it works
Puppeteer is a Node.js library that drives a headless (or full) instance of Chromium. You load your HTML either via page.goto(url)
or page.setContent(htmlString)
then call page.pdf()
to capture a PDF snapshot of exactly what the browser renders.
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.setContent('<h1>Invoice #123</h1><p>...</p>');
await page.pdf({
path: 'invoice.pdf',
format: 'A4',
printBackground: true,
margin: { top: '20mm', bottom: '20mm' }
});
await browser.close();
})();
Pros
- Full modern-browser fidelity: Supports CSS3, web fonts, JavaScript, SVG, and complex layouts.
- Fine-grained control: You can inject scripts, wait for network idle, manipulate the DOM, and toggle “print” CSS before rendering.
Cons
- High startup cost: Launching Chrome is heavy – hundreds of megabytes of RAM and nontrivial CPU even before rendering.
- Concurrency management: To generate many PDFs in parallel, you must manage a pool of browser instances or reuse pages, or risk overwhelming your server.
- Maintenance overhead: You’re responsible for updating Chromium, handling version mismatches, and troubleshooting headless-specific quirks.
You can find out more in the following article:
wkhtmltopdf (WebKit-Based CLI)
wkhtmltopdf
is a standalone binary that uses the Qt WebKit engine to convert HTML files or URLs directly into PDFs:
wkhtmltopdf --print-media-type \
--margin-top 15mm \
--margin-bottom 15mm \
invoice.html invoice.pdf
Pros
- Lightweight binary: No need to run a full browser – just install the
wkhtmltopdf
package. - Basic features: Supports headers/footers, table of contents, and simple CSS print rules.
Cons
- Outdated engine: Lacks support for modern CSS (flexbox, grid) and has limited JavaScript execution: dynamic content often doesn’t render correctly.
- Dependency headaches: Requires specific system libraries (Qt, fontconfig) and can be tricky to install in containerized or minimal OS environments.
- Limited layout control: Advanced pagination tricks (avoiding orphans/widows, complex page-break logic) often require cumbersome CSS hacks or aren’t supported.
Other Open-Source Libraries and Tools
Beyond Puppeteer and wkhtmltopdf, there are other tools worth mentioning:
- WeasyPrint: A robust Python library that converts HTML and CSS to PDF, focusing on compliance with print CSS standards. WeasyPrint excels at handling complex layouts and modern CSS (better than wkhtmltopdf in many cases) and is great if you’re in a Python ecosystem. However, it does not support JavaScript in the HTML at all, so dynamic graphs or client-side formatting won’t work – it’s for static HTML/CSS conversion only.
- Headless Chrome via other frameworks: Puppeteer is one option; Playwright is another Node.js library similar to Puppeteer that can use not just Chrome but also Firefox and WebKit engines. Electron (the framework behind many desktop apps) also has the ability to generate PDFs from a headless Chromium instance. These all provide modern rendering but come with the same overhead of running a browser.
- jsPDF and PDF libraries: There are libraries like jsPDF (for JavaScript) or iText (Java/.NET), but these generate PDFs by constructing them programmatically rather than converting HTML. They are useful for simple documents or if you cannot use HTML, but if you already have an HTML invoice template designed, these require reimplementing the layout in code, which is cumbersome.
In summary, developers have no shortage of open-source solutions to tackle HTML to PDF. If you’re comfortable coding and managing infrastructure, you can get good results with these tools.
However, as your eCommerce business or application grows, you might start to hit the limitations of maintaining these yourself.
3. Benefits of Automated HTML-to-PDF Generation APIs
Shifting from self-hosted converters to a managed API for HTML-to-PDF brings a suite of advantages that directly address the common pain points of DIY approaches:
- Zero Infrastructure Overhead: You no longer need to install headless browsers, maintain wkhtmltopdf binaries, or orchestrate container clusters just for PDF rendering. A single HTTP request to the API and APITemplate.io handles the heavy lifting.
- Instant Scalability & Burst Handling: Managed APIs elastically scale to meet demand. Whether you’re generating ten labels per minute or ten thousand invoices during a flash sale, the service provisions the required compute under the hood. Many APIs including APITemplate.io offer asynchronous rendering with webhooks, so you can fire off bulk jobs without blocking your application.
- Browser-Level Fidelity: Advanced CSS, web fonts, and client-side JavaScript are no longer second-class citizens.
- Built-In, Advanced PDF Features: Toggling on page headers/footers, dynamic page-numbering, custom page sizes, watermarks, or table-of-contents generation is handled via simple API parameters or template settings.
- Enterprise-Grade Reliability & Support: APIs come with SLAs, monitoring, and support teams. Should a conversion error occur or a regional outage threaten your workflow, you can rely on status pages, alerts, and direct assistance. This peace of mind is critical for eCommerce operations during peak shopping periods. You can learn more about the enterprise features here.
- Accelerated Development & Reduced Maintenance: By outsourcing the nuances of PDF generation, your engineers spend less time wrestling with rendering edge-cases and more time on core business features.
- Support of Nocode Platforms: APITemplate.io offers integrations with Zapier, Make.com, Bubble.io and some other popular no-code platforms.
Of course, one must consider things like cost (API services usually have usage-based pricing) and ensuring you trust the provider with your data (since you’ll be sending order info to them).
Many services address privacy by offering data region isolation or even on-premise options. In the case of APITemplate.io, they provide regional endpoints (US, EU, Asia, etc.) to keep data in-region for compliance. And there’s usually a free tier, so you can try it out with no commitment.
Speaking of which, let’s dive into APITemplate.io specifically – a popular API-based HTML to PDF generator – and see what it offers, especially for eCommerce scenarios.
4. APITemplate.io: HTML to PDF Generation Made Easy
When it’s time to move beyond self-hosted converters and offload the heavy lifting of PDF generation, APITemplate.io offers a turnkey, developer-centric platform.
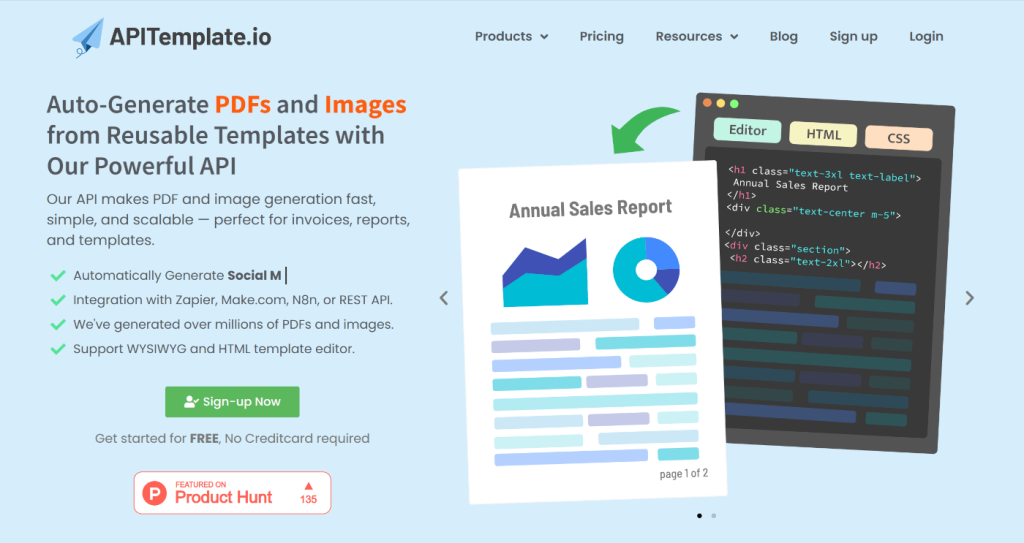
Rather than wrestling with browser binaries or CLI quirks, you get a managed service that handles everything from rendering to scaling, so you can focus on your eCommerce logic.
- Visual & Code-First Template Designer: APITemplate.io’s template designer lets you build layouts either by dragging and dropping elements in a WYSIWYG interface or by editing HTML and CSS directly. A real-time preview shows exactly how each page, header, and footer will appear in the final PDF.
- Template-Driven Data Binding: You create a single template (for example, a 4×6 shipping label) and insert placeholders like
{{sku}}
or{{barcode_url}}
. Each API call simply supplies a JSON payload to populate those fields, keeping design and data neatly separated. - Developer-Friendly Integration: We offer PDF generation API, call a single endpoint from any language (Node.js, Python, PHP, Java, C#, etc.). In addition, APITemplate.io supports Webhook & Async Mode, for high-volume or large PDFs, submit requests asynchronously and receive a callback when ready.
- Modern Rendering Engine: Under the hood, the service uses a current browser engine that fully supports HTML5, CSS3, and client-side JavaScript. This ensures your templates render in PDF exactly as they do in a browser, including custom fonts and dynamic charts.
- Built-In PDF Features: APITemplate.io automatically handles page numbering (e.g., “Page 1 of 3”), repeating headers and footers, and table-of-contents generation without extra code. You can set page size, orientation, and margins directly in the template settings.
- Scalable, High-Performance Infrastructure: Regional endpoints in the US, EU, Singapore, and Australia reduce latency and support data-residency requirements. The platform elastically scales from single PDF requests to high-volume label batches without any infrastructure changes on your side.
- Security & Compliance: All traffic is encrypted over HTTPS and authenticated via API keys. You can choose regional endpoints to keep data within specific jurisdictions and generate expiring download links or have PDFs emailed directly to end users.
- Flexible Pricing & Trial: A generous free tier lets you experiment with templates and low-volume use cases at no cost. Usage-based plans scale predictably with your order volume, and enterprise options provide dedicated throughput and SLAs.
By abstracting away infrastructure, updates, and edge-case rendering issues, APITemplate.io empowers you to deliver pixel-perfect eCommerce documents: receipts, labels, invoices – faster and with far less overhead than DIY solutions.
5. Steps to Create PDF Labels with APITemplate.io
Label generation is a perfect starter project for APITemplate.io’s template-plus-data workflow. The walkthrough below shows how to design a Avery label sheets 5160, render it via the v2 API and retrieve the finished PDF.
Step 1: Design your label template
- Log in to APITemplate.io and navigate to Manage Templates → New Template, then choose a template to start with.
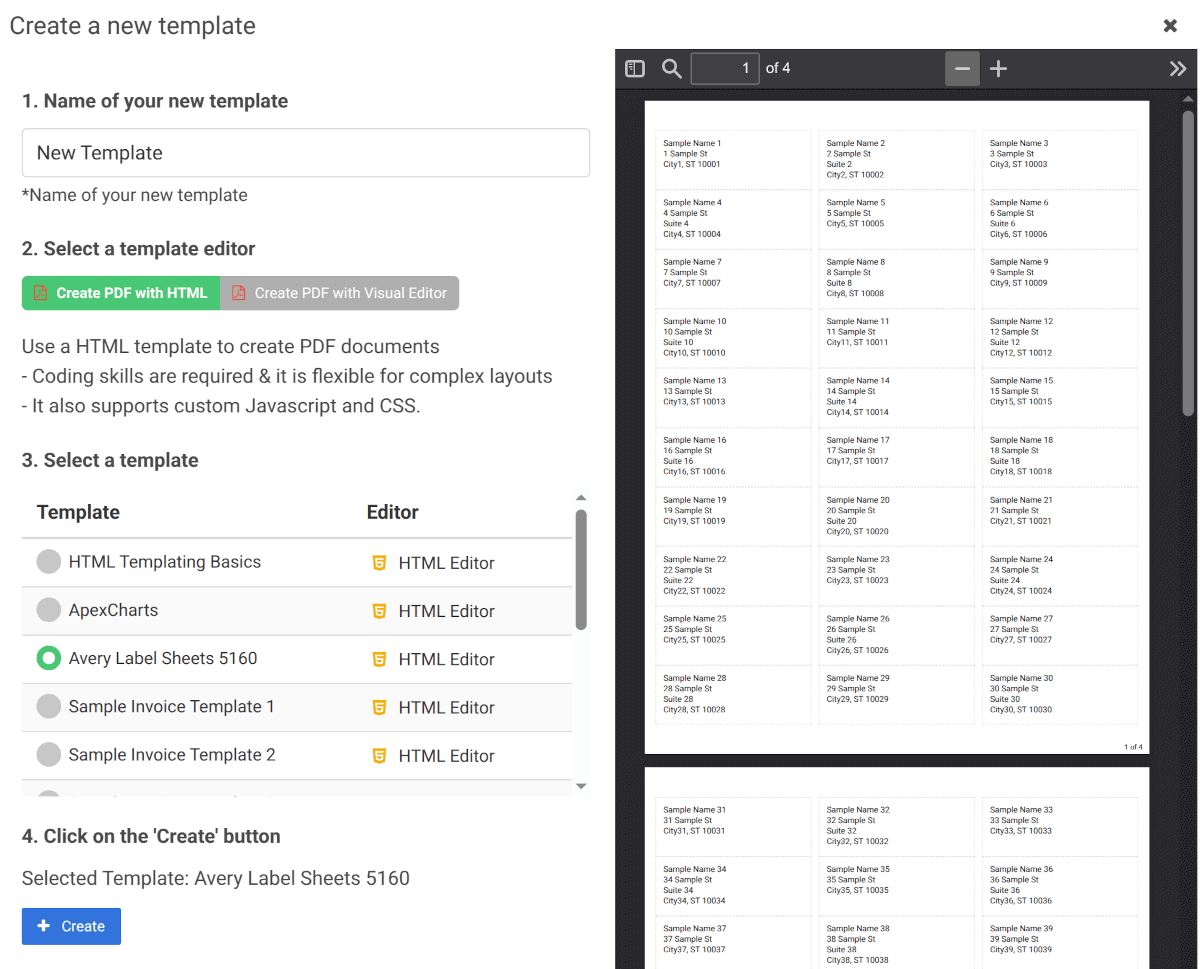
- Choose PDF and switch to the HTML/CSS editor (or use the WYSIWYG mode).
- Lay out your label and insert dynamic placeholders such as
{{name}}
,{{address_line1}}
,{{
2address_line
}}
,{{city}}
etc. - In Settings, set the page size to Letter and adjust margins to suit your label printer.
Step 2: Preview and save
- Click Quick Preview or Generate PDF to verify your design.
- Press Save to save the template
Step 3: Prepare your JSON payload
{
"labels": [
{
"name": "Sample Name 1",
"address_line1": "1 Sample St",
"address_line2": null,
"city": "City1",
"state": "ST",
"zip": "10001"
},
{
"name": "Sample Name 2",
"address_line1": "2 Sample St",
"address_line2": "Suite 2",
"city": "City2",
"state": "ST",
"zip": "10002"
},
{
"name": "Sample Name 3",
"address_line1": "3 Sample St",
"address_line2": null,
"city": "City3",
"state": "ST",
"zip": "10003"
}
]
}
Step 4: Call the PDF render endpoint
Use curl
, Postman or your favorite HTTP client:
curl --header "Content-Type: application/json" \
-H 'X-API-KEY: 6fa6g2pdXGIyHRhVlGh7U56Ada1eF' \
--data '{"labels":[{"name":"Sample Name 1","address_line1":"1 Sample St","address_line2":null,"city":"City1","state":"ST","zip":"10001"},{"name":"Sample Name 2","address_line1":"2 Sample St","address_line2":"Suite 2","city":"City2","state":"ST","zip":"10002"},{"name":"Sample Name 3","address_line1":"3 Sample St","address_line2":null,"city":"City3","state":"ST","zip":"10003"}]}' \
"https://rest.apitemplate.io/v2/create-pdf?template_id=79667b2b1876e347"
The JSON response contains a download_url
and your ready-to-print PDF label.
Step 5: Download or stream the PDF
- Fetch the
download_url
in your backend, or pass it directly to a label-printing microservice. - For bulk runs, fire multiple requests in parallel or use APITemplate.io’s async webhook mode to receive callbacks as each PDF finishes.
API Reference.
Grab the full OpenAPI v2 specification: the complete with schemas, parameters and error codes here:
https://apitemplate.io/apiv2/
With a reusable template, a simple JSON payload and one POST request, you can weave PDF label generation into any eCommerce workflow – no browser automation, no server headaches.
6. Open-Source vs. APITemplate.io: Feature Comparison
To summarize the differences between managing HTML-to-PDF conversion yourself (with open-source tools) and using APITemplate.io, here’s a streamlined comparison:
Aspect | Open-Source Tools | APITemplate.io API |
---|---|---|
Setup & Maintenance | You install, configure and update binaries or runtimes yourself. | Zero setup and just call the API; all updates handled by APITemplate.io. |
Ease of Use | Requires custom scripts, local template management, and ops work. | Simple REST/SDK integration and web-based template editor. |
Rendering Fidelity | Varies by engine and older tools miss modern CSS/JS, headless Chrome is heavy. | Modern browser engine under the hood; full HTML/CSS/JS support. |
Scalability | You must provision and orchestrate servers or browser instances to handle load. | Cloud-scale rendering with sync or async (webhook) modes and no infra to manage. |
Advanced Features | Headers/footers, pagination, and template logic need manual implementation. | Built-in pagination, page numbering, headers/footers and data binding in templates. |
Cost | “Free” software but hidden DevOps and maintenance costs. | Usage-based pricing with a free tier; saves engineering time and infrastructure spend. |
As shown above, open-source tools offer full control and zero license fees but require ongoing maintenance and scaling effort, whereas APITemplate.io delivers a turnkey, feature-rich PDF service that lets your team focus on core eCommerce logic rather than PDF infrastructure.
7. Conclusion & Next Steps
HTML-to-PDF conversion is the backbone of eCommerce paperwork. You can build it yourself, but you’ll juggle headless browsers, server spikes and endless CSS quirks.
Or you can outsource the grunt work to a specialized API like APITemplate.io, gaining instant scalability, designer-friendly templates and modern rendering – all with a couple of HTTP requests.
Ready to try it? Sign up for APITemplate.io’s free tier, design your first label or invoice and integrate the API in minutes. Your customers get professional, printer-perfect PDFs – you get more time to grow your store.